Well well, after programming so much with out OOP, when we learned it, it actually made so much more sense to program with it. So instead of every function being it’s own “being”, we put the functions and variables assosiated with one “concept” in one class, and when you need to use that function again and again all you need to do is create an instance of that class by saying $instance_name = new class_name();
And choosing which function or variable you like to use in that specific class with a “->”. It’s that simple!
So here I practiced my OOP with creating a application which allows users to either log in or register, after logged on they are taken to a profile page where they see two lists a list of other names of people that are registered and a list of those that befriended them. They have the option of befriending new friends by clicking on the button near their names in the registered list, and automatically the list of friends will update itself and the button in the registered list will turn in to the word that says “Friends”.
The register/login page: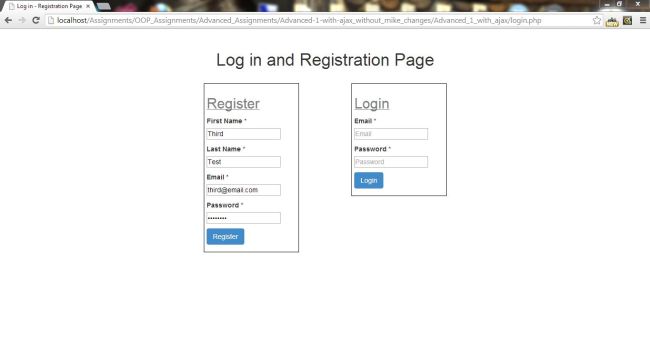
The profile page: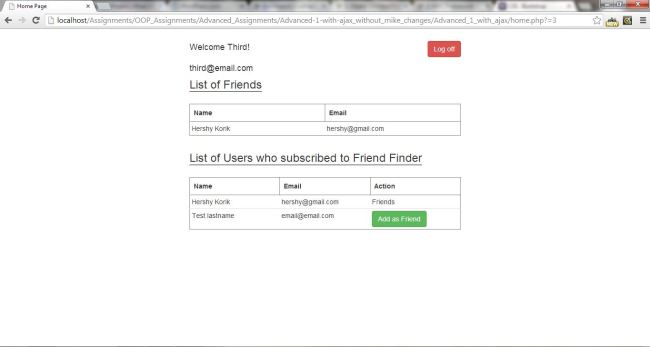
Github Source Code:
https://github.com/hkorik/OOP_Project.git
login.php Code:
<?php
include("connection.php");
if(isset($_SESSION['logged_in']))
{
header("Location: home.php");
}
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Log in - Registration Page</title>
<link rel="stylesheet" type="text/css" href="CSS/styles.css" />
<link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css">
</head>
<body>
<div id="wrapper">
<h1>Log in and Registration Page</h1>
<!-- Registration left box -->
<div id="registration_box" class="float_left">
<h2>Register</h2>
<?php
if(isset($_SESSION['register_errors']))
{
foreach($_SESSION['register_errors'] as $error)
{
echo "<p class='error_message'>$error</p>";
}
}
else if(isset($_SESSION['register_message']))
{
echo "<p class='success_message'>{$_SESSION['register_message']}</p>";
unset($_SESSION['register_message']);
}
?>
<form action="process.php" method="post">
<input type="hidden" name="action" value="register" />
<div class="<?php if(isset($_SESSION['register_errors']['first_n_error'])) echo 'field_block'; ?>">
<label for="first_name">First Name *</label><br/>
<input type="text" name="first_name" id="first_name" placeholder="First Name" />
</div>
<div class="<?php if(isset($_SESSION['register_errors']['last_n_error'])) echo 'field_block'; ?>">
<label for="last_name">Last Name *</label><br/>
<input type="text" name="last_name" id="last_name"placeholder="Last Name" />
</div>
<div class="<?php if(isset($_SESSION['register_errors']['email_error'])) echo 'field_block'; ?>">
<label for="email">Email *</label><br/>
<input type="text" name="email" id="email" placeholder="Email" />
</div>
<div class="<?php if(isset($_SESSION['register_errors']['pw_error'])) echo 'field_block'; ?>">
<label for="password">Password *</label><br/>
<input type="password" name="password" id="password" placeholder="Password" />
</div>
<input class="btn btn-primary" type="submit" value="Register" />
</form>
<?php unset($_SESSION['register_errors']); ?>
</div>
<!-- Login right box -->
<div id="login_box" class="float_right">
<h2>Login</h2>
<?php
if(isset($_SESSION['login_errors']))
{
foreach($_SESSION['login_errors'] as $error)
{
echo "<p class='error_message'>$error</p>";
}
}
else if(isset($_SESSION['message']))
{
}
?>
<form action="process.php" method="post">
<input type="hidden" name="action" value="login" />
<div class="<?php if(isset($_SESSION['login_errors']['email_error'])) echo 'field_block'; ?>">
<label for="email">Email *</label><br/>
<input type="text" name="email" id="email" placeholder="Email" />
</div>
<div class="<?php if(isset($_SESSION['login_errors']['pw_error'])) echo 'field_block'; ?>">
<label for="password">Password *</label><br/>
<input type="password" name="password" id="password" placeholder="Password" />
</div>
<input class="btn btn-primary" type="submit" value="Login" />
</form>
<?php unset($_SESSION['login_errors']); ?>
</div>
</div>
</body>
</html>
home.php Code:
<?php
include("connection.php");
if(!isset($_SESSION['logged_in']))
{
header("Location: login.php");
}
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home Page</title>
<link rel="stylesheet" type="text/css" href="CSS/styles.css" />
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
<link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css">
<link rel="stylesheet" media="all" type="text/css" href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.16/themes/ui-lightness/jquery-ui.css" />
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.16/jquery-ui.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('#user_form').submit(function(){
var form = $(this);
$.post(
form.attr('action'),
form.serialize(),
function(data){
$('#users_list').html(data);
},
'json');
return false;
});
$('#user_form').submit();
$('#friend_form').submit(function(){
var form = $(this);
$.post(
form.attr('action'),
form.serialize(),
function(data){
$('#friends_list').html(data);
},
'json');
return false;
});
$('#friend_form').submit();
$(document).on('submit', '.add_friend_form', function(){
var form = $(this);
$.post(
form.attr('action'),
form.serialize(),
function(data){
$('#user_form').submit();
$('#friend_form').submit();
},
'json');
return false;
});
});
</script>
</head>
<body>
<div id="wrapper_home">
<div id="header">
<p class="float_left">Welcome <?php echo "{$_SESSION['user']['first_name']}" ?>!</p>
<p class="float_right"><a class="btn btn-danger" href="process.php">Log off</a></p>
<p class="clear"><?php echo "{$_SESSION['user']['email']}" ?></p>
</div>
<div id="main">
<h3>List of Friends</h3>
<form id="friend_form" action="process.php" method="post">
<input type="hidden" name="action" value="friends" />
</form>
<table class="table">
<thead>
<th>Name</th>
<th>Email</th>
</thead>
<tbody id="friends_list">
</tbody>
</table>
<h3>List of Users who subscribed to Friend Finder</h3>
<form id="user_form" action="process.php" method="post">
<input type="hidden" name="action" value="users" />
</form>
<table class="table">
<thead>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</thead>
<tbody id="users_list">
</tbody>
</table>
</div>
</div>
</body>
</html>
styles.css Code:
*{
margin: 0px;
padding: 0px;
}
.float_left{
float:left;
}
.float_right{
float:right;
}
.clear{
clear: both;
}
#wrapper{
padding: 15px;
width:600px;
margin: 10px auto;
}
#wrapper h1{
text-align: center;
}
/*Pop up Messages styling*/
.success_message{
color: green;
}
.error_message{
color: red;
}
.highlighted{
border:1px solid red;
}
.field_block{
border:1px solid red;
width:160px;
margin-bottom:5px;
}
/* Registration left box*/
#registration_box {
border:1px solid black;
padding: 5px;
margin-top: 20px;
margin-left: 30px;
width:200px;
}
#registration_box h2{
color:grey;
text-decoration: underline;
}
#registration_box form{
margin-top:10px;
}
#registration_box input{
margin-bottom: 10px;
}
/* Login right box*/
#login_box {
border:1px solid black;
padding: 5px;
margin-top: 20px;
margin-right: 30px;
width:200px;
}
#login_box h2{
color:grey;
text-decoration: underline;
}
#login_box form{
margin-top:10px;
}
#login_box input{
margin-bottom: 10px;
}
#wrapper_home{
padding: 15px;
width:600px;
margin: 10px auto;
}
#wrapper_home #header{
height: 40px;
}
#wrapper_home #header p{
font-size: 18px;
}
#wrapper_home #main{
margin: 20px 0px 0px 0px;
}
#wrapper_home #main h3{
border-bottom: 1px solid black;
display: inline-block;
}
#wrapper_home #main table{
margin: 15px 0px;
border: 1px solid grey;
}
#wrapper_home #main th{
border: 1px solid grey;
width:150px;
}
#wrapper_home #main td{
padding: 4px;
}
constant.php Code:
<?php
//define constants for db_host, db_user, db_pass, and db_database
//adjust the values below to match your database settings
define('DB_HOST', 'localhost');
define('DB_USER', 'root');
define('DB_PASS', ''); //set DB_PASS as 'root' if you're using MAMP
define('DB_DATABASE', 'friends');
?>
connection.php Code:
<?php
include("constant.php");
session_start();
class Database{
public function __construct()
{
//connect to database host
$connection = mysql_connect(DB_HOST, DB_USER, DB_PASS) or die('Could not connect to the database host (please double check the settings in connection.php): ' . mysql_error());
//connect to the database
$db_selected = mysql_select_db(DB_DATABASE, $connection) or die ('Could not find a database with the name "'.DB_DATABASE.'" (please double check your settings in connection.php): ' . mysql_error());
}
//fetches all records from the query and returns an array with the fetched records
function fetch_all($query)
{
$data = array();
$result = mysql_query($query);
while($row = mysql_fetch_assoc($result))
{
$data[] = $row;
}
return $data;
}
//fetch the first record obtained from the query
function fetch_record($query)
{
$result = mysql_query($query);
return mysql_fetch_assoc($result);
}
}
?>
process.php Code:
<?php
include("connection.php");
class Process{
var $run_database;
var $html;
var $table1;
function __construct()
{
$this->run_database = new Database();
//When user registers - run register function
if(isset($_POST['action']) and $_POST['action'] == "register")
{
$this->register_action();
}
//When user logs in - run login function
else if (isset($_POST['action']) and $_POST['action'] == "login")
{
$this->login_action();
}
elseif(isset($_POST['action']) and $_POST['action'] == "friends")
{
$this->get_friends_list();
$data = $this->table1;
echo json_encode($data);
}
elseif(isset($_POST['action']) and $_POST['action'] == "users")
{
$this->get_users_list();
$data = $this->html;
echo json_encode($data);
}
else if(isset($_POST['action']) and $_POST['action'] == "users_id")
{
$this->add_friend();
$added = "added friend";
echo json_encode($added);
}
else
{
session_destroy();
header("Location: login.php");
}
}
function register_action()
{
$errors = NULL;
//Email validation
if(empty($_POST['email']))
{
$errors['email_error'] = "Error: Email address cannot be blank!";
}
else if(!filter_var($_POST['email'], FILTER_VALIDATE_EMAIL))
{
$errors['email_error'] = "Error: Email should be in valid format!";
}
//First name validation
if(empty($_POST['first_name']))
{
$errors['first_n_error'] = "Error: First name field cannot be blank!";
}
else if (preg_match('#[0-9]#', $_POST['first_name']))
{
$errors['first_n_error'] = "Error: First name cannot contain numbers!";
}
//Last name validation
if(empty($_POST['last_name']))
{
$errors['last_n_error'] = "Error: Last name field cannot be blank!";
}
else if (preg_match('#[0-9]#', $_POST['last_name']))
{
$errors['last_n_error'] = "Error: Last name cannot contain numbers!";
}
//Password validation
if(empty($_POST['password']))
{
$errors['pw_error'] = "Error: Password field cannot be blank!";
}
else if(strlen($_POST['password']) < 6)
{
$errors['pw_error'] = "Error: Password must be greater than 6 charecters";
}
//if there are any errors
if(count($errors) > 0)
{
$_SESSION['register_errors'] = $errors;
header("Location: login.php");
}
// if everything is correct!
else
{
//check if user email exists
$check_user = "SELECT * FROM users WHERE email = '{$_POST['email']}'";
$user_exist = $this->run_database->fetch_record($check_user);
// if no one has that email address
if($user_exist == NULL)
{
$new_user = "INSERT INTO users (first_name, last_name, email, password, created_at) VALUES ('{$_POST['first_name']}','{$_POST['last_name']}','{$_POST['email']}','" . md5($_POST['password']) . "', now() )";
mysql_query($new_user);
$check_user_info = "SELECT * FROM users WHERE email = '{$_POST['email']}' AND password = '" . md5($_POST['password']) . "'";
$user_info = $this->run_database->fetch_record($check_user_info);
$_SESSION['user']['id'] = $user_info['id'];
$_SESSION['user']['first_name'] = $user_info['first_name'];
$_SESSION['user']['last_name'] = $user_info['last_name'];
$_SESSION['user']['email'] = $user_info['email'];
$_SESSION['logged_in'] = TRUE;
if($_SESSION['logged_in'] == TRUE)
{
header("Location: home.php?=" . $_SESSION['user']['id']);
}
}
// if email already exists
else
{
$errors['email_error'] = "Error: Email {$_POST['email']} is already in use!";
$_SESSION['register_errors'] = $errors;
header("Location: login.php");
}
}
}
//login button function
function login_action()
{
$errors_login = NULL;
//Email validation
if(empty($_POST['email']))
{
$errors_login['email_error'] = "Error: Email address cannot be blank!";
}
else if(!filter_var($_POST['email'], FILTER_VALIDATE_EMAIL))
{
$errors_login['email_error'] = "Error: Email should be in valid format!";
}
//Password validation
if(empty($_POST['password']))
{
$errors_login['pw_error'] = "Error: Password field cannot be blank!";
}
else if(strlen($_POST['password']) < 6)
{
$errors_login['pw_error'] = "Error: Password length must be at least 6 charecters!";
}
//if there are any error
if(count($errors_login) > 0)
{
$_SESSION['login_errors'] = $errors_login;
header("Location: login.php");
}
// if everything is correct!
else
{
$check_user_info = "SELECT * FROM users WHERE email = '{$_POST['email']}' AND password = '" . md5($_POST['password']) . "'";
$user_info = $this->run_database->fetch_record($check_user_info);
if($user_info != NULL)
{
$_SESSION['user']['id'] = $user_info['id'];
$_SESSION['user']['first_name'] = $user_info['first_name'];
$_SESSION['user']['last_name'] = $user_info['last_name'];
$_SESSION['user']['email'] = $user_info['email'];
$_SESSION['logged_in'] = TRUE;
if($_SESSION['logged_in'] == TRUE)
{
header("Location: home.php?=" . $_SESSION['user']['id']);
}
}
else
{
$errors[] = "Error: The information entered does not match any of our records!";
$_SESSION['login_errors'] = $errors;
header("Location: login.php");
}
}
}
function get_users_list()
{
$friend_status = array();
// query to get all the friends
$friends_query = "SELECT * FROM friends";
$friends = $this->run_database->fetch_all($friends_query);
// check if the current user's id exists in the friends table
foreach($friends as $friend)
{
if($_SESSION['user']['id'] == $friend['user_id'])
{
$friend_status[$friend['friend_id']] = TRUE;
}
}
// store it in a another array $friend_status
$users_query = "SELECT id, first_name, last_name, email
FROM users
WHERE users.id != '{$_SESSION['user']['id']}'";
$users_list = $this->run_database->fetch_all($users_query);
foreach($users_list as $user)
{
$this->html .= "<tr>";
$this->html .= "<td>{$user['first_name']} {$user['last_name']}</td>
<td>{$user['email']}</td>";
if(isset($friend_status[$user['id']]))
{
$this->html .= "<td>Friends</td>";
}
else
{
$this->html .= "<td>
<form class='add_friend_form' action='process.php' method='post'>
<input type='hidden' name='action' value='users_id'>
<input type='hidden' name='user_id' value='{$user['id']}'>
<input class='btn btn-success' type='submit' value='Add as Friend' />
</form>
</td>";
}
$this->html .= "</tr>";
}
}
function get_friends_list()
{
$query = "SELECT users.first_name, users.last_name, users.email
FROM users
LEFT JOIN friends
ON friends.friend_id = users.id
WHERE friends.user_id = '{$_SESSION['user']['id']}'";
$friends_list = $this->run_database->fetch_all($query);
foreach($friends_list as $friend)
{
$this->table1 .= "<tr>
<td>{$friend['first_name']} {$friend['last_name']}</td>
<td>{$friend['email']}</td></tr>";
}
}
function add_friend()
{
$query = "INSERT INTO friends (user_id, friend_id) VALUES ('{$_SESSION['user']['id']}', '{$_POST['user_id']}'), ('{$_POST['user_id']}', '{$_SESSION['user']['id']}')";
mysql_query($query);
}
}
$process = new Process();
?>